In This Topic
Barcodes in Nevron Open Vision (NOV) are implemented as widgets, so you can easily embed them in your NOV based applications, rich text documents and reports.
Barcodes in Applications
To show a linear or a 2D barcode in your application you should simply create a barcode widget, set its properties and place it somewhere in you application. The following code demonstrates how to create and initialize an EAN13 linear barcode and place it in a stack panel:
Linear Barcode Widget |
Copy Code
|
NLinearBarcode barcode = new NLinearBarcode();
barcode.HorizontalPlacement = ENHorizontalPlacement.Center;
barcode.VerticalPlacement = ENVerticalPlacement.Center;
barcode.Symbology = ENLinearBarcodeSymbology.EAN13;
barcode.Text = "0123456789012";
stackPanel.Add(barcode);
|
This code results in the following barcode:
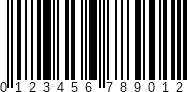
Barcodes as Images
Nevron Open Vision's barcode library lets you easily create barcode images by using the proper barcode painter class. For example the code below creates a 2D barcode image by the QR code standard:
QR Code Image |
Copy Code
|
NMatrixBarcodePainter painter = new NMatrixBarcodePainter();
painter.Symbology = ENMatrixBarcodeSymbology.QrCode;
painter.Text = "https://www.nevron.com";
NRaster qrRaster = painter.CreateRaster(100, 100, NRaster.DefaultResolution);
NImage qrImage = new NImage(qrRaster);
|
This code results in the following QR code image:
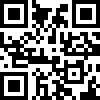
Barcodes in Text Documents
You can easily insert barcodes in rich text documents. All you have to do is to create and initialize a barcode widget and then create and add a widget inline to a paragraph of the rich text document. As all other widgets barcode widgets used in rich text documents are automatically converted to images when saving the document to a text format not native to NOV Text Editor (DOCX, HTML, RTF, etc.).
Barcode in a Rich Text Document |
Copy Code
|
// Create a barcode widget
NBarcode barcode = new NLinearBarcode(ENLinearBarcodeSymbology.EAN13,"0123456789012");
NWidgetInline widgetInline = new NWidgetInline(barcode);
// Add the linear barcode widget inline to a paragraph
paragraph.Inlines.Add(widgetInline);
|
Barcodes in Diagrams
You can easily insert barcodes in Nevron Diagram shapes. All you have to do is to create and initialize a barcode widget and set it to the Widget property of a shape:
Barcode in a Diagram Shape |
Copy Code
|
// Create a barcode widget
NMatrixBarcode barcode = new NMatrixBarcode(ENMatrixBarcodeSymbology.QrCode, "https://www.nevron.com");
// Create a shape and place the barcode widget in it
NShape shape = new NShape();
shape.SetBounds(100, 100, 100, 100);
shape.Widget = barcode;
activePage.Items.Add(shape);
|
See Also